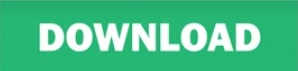
On line #7 of the example above, the object named dice with the type Random is declared.You can also create random numbers by using the Random class of the Java API library.Objective #2: Use the Random class for random number generation to create simulations. You will not be tested on the information below and it is not covered on the AP Computer Science exam. Int num = (int) (Math.random() * 2) + 1 // 1 for tails, 2 for heads Int count = 0 // number of consecutive heads Here's a method that uses a flag variable to count the number of consecutive flips of heads on a coin:.Public static boolean willItSnowOrNot(double percentage) Or this version that accepts a double parameter instead of an int Public static boolean willItSnowOrNot(int percent) This example, accepts a parameter percent and returns a true percent% of the time. You can generate and return a boolean value based on a desired random percent or percentage.You can also generate and return boolean values instead of integers.Public static int rollDice(int low, int high) Return (int) (Math.random() * (high - low + 1)) + low
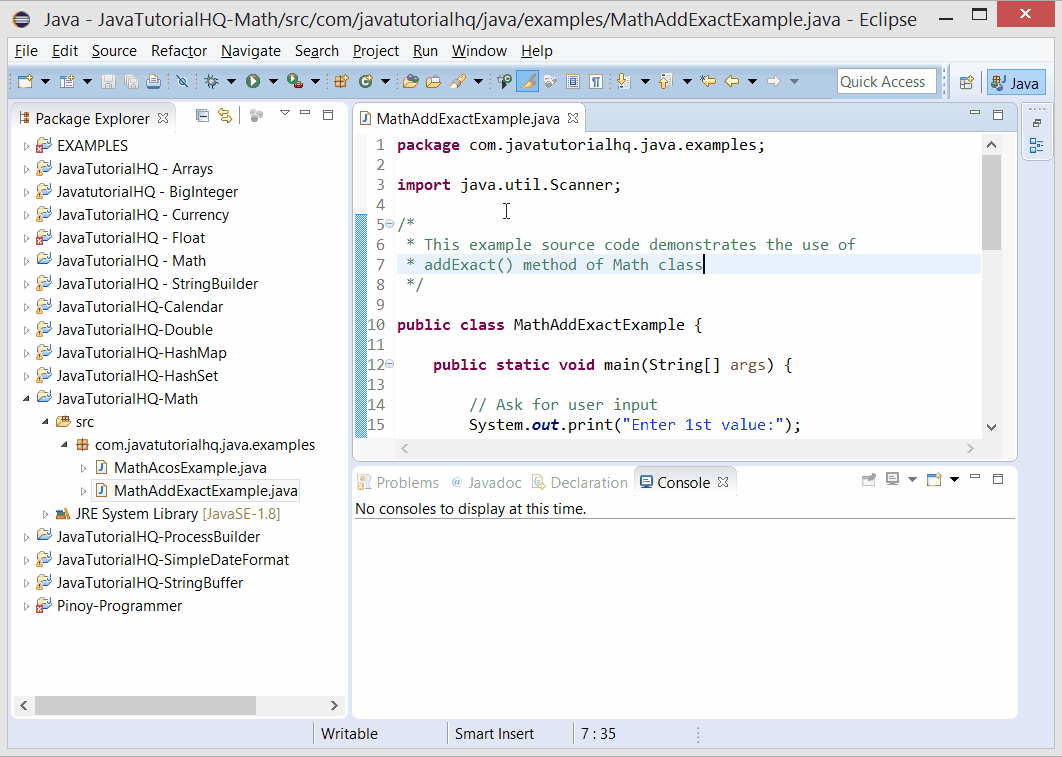
Public static int generateRandom(int low, int high) Here is a method that generates a random whole number between two positive integers.Here is a method that accepts parameters to simulate a dice roll where the dice has.You can create reusable methods that generate random values.Objective #2: Use the Math.random static method in methods.
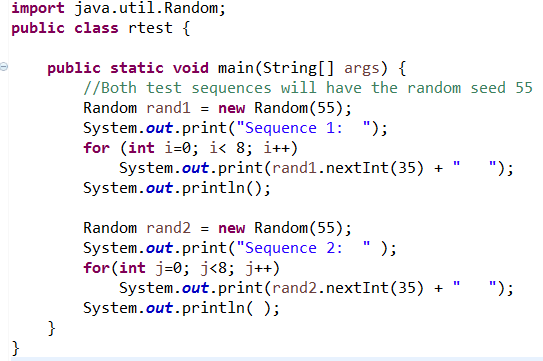
Int prob = Math.floor(Math.random() * 3) The method should be written the following way: The problem is that Math.random is being called twice and the conditional probability of a lose is not 1/3. Write a method that returns "win", "lose", or "draw" each 1/3 of the time.Įlse if (Math.floor(Math.random() * 3 = 1)
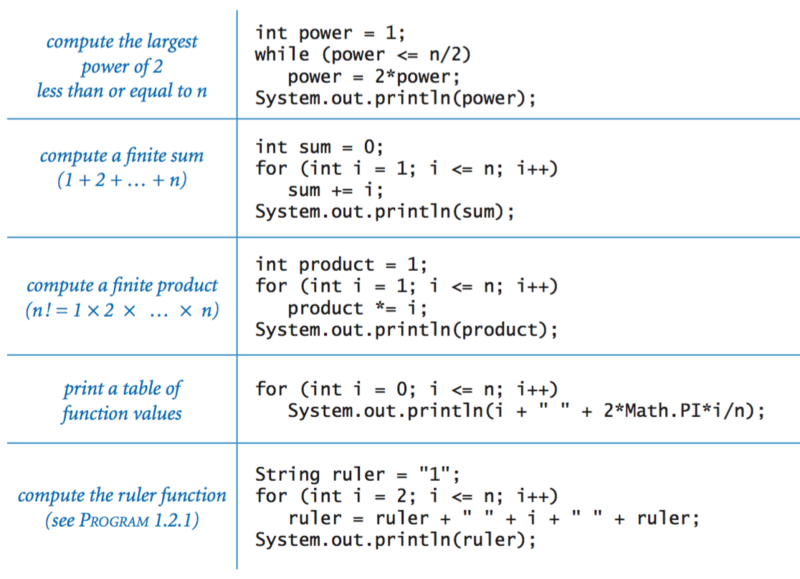
Num = (int) (Math.random() * 10) - 4 // -4 <= num <= 5īe careful to use the extra set of parentheses in the examples above since Num = (int) (Math.random() * 2) + 1 // 1 or 2 (simulated coin flip) By typecasting the result to an integer and truncating the decimal places, we can ensure that only whole numbers are produced as in:.You can manipulate this returned value by turning it into an integer or "spreading out" the range of possible values.The static method named random in the Math class can be used to create a random decimal value that is greater than or equal to 0.0 and less than 1.0.įor example, the following statement displays a random decimal value such as 0.34.So it is proper to call these values pseudorandom rather than random. It is technically impossible to create a truly random value with a computer since any available built-in method or process for creating a random value relies on some determinate mechanism such as the computer's system clock.Objective #1: Use the Math.random static method for random number generation to create
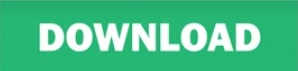